728x90
1. LinkedListstack.h
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
|
#include <string.h>
#include <stdlib.h>
#include <stdio.h>
typedef struct Node
{
char *Data;
struct Node* Next;
}Node;
typedef struct LinkedStack
{
Node* List;
Node* Top;
}LLS;
Node* LLS_CreateStack(LLS** Stack);
Node* LLS_CreateNode(char* newData);
void LLS_DestroyNode(Node* _Node);
void LLS_Push(LLS* Stack, Node* NewNode);
Node* LLS_Pop(LLS* Stack);
Node* LLS_Top(LLS* Stack);
int LLS_GetSize(LLS* Stack);
int LLS_IsEmpaty(LLS* Stack);
typedef LLS LinkedStack;
Node* LLS_CreateStack(LLS** Stack)
{
(*Stack) = (LLS*)malloc(sizeof(LLS));
(*Stack)->List = NULL;
(*Stack)->Top = NULL;
}
Node* LLS_CreateNode(char* newData)
{
unsigned short str_length = strlen(newData) + 1;
Node* NewNode = (Node*)malloc(sizeof(NewNode));
NewNode->Data = (char*)malloc(str_length);
strcpy_s(NewNode->Data, str_length, newData);
NewNode->Next = NULL;
return NewNode;
}
int LLS_IsEmpaty(LLS* Stack)
{
return (Stack->List == NULL);
}
void LLS_DestroyNode(Node* _Node)
{
free(_Node->Data);
free(_Node);
}
Node* LLS_Pop(LLS* Stack)
{
//함수가 반환할 최상위 노드
Node* TopNode = Stack->Top;
if (Stack->List == Stack->Top)
{
Stack->List = NULL;
Stack->Top = NULL;
}
else
{
/*새로운 최상위 노드를 스택의 Top 필드에 등록*/
Node* CurrentTop = Stack->List;
while (CurrentTop != NULL && CurrentTop->Next != Stack->Top)
{
CurrentTop = CurrentTop->Next;
}
Stack->Top = CurrentTop;
CurrentTop->Next = NULL;
}
return TopNode;
}
void LLS_Push(LLS* Stack, Node* NewNode)
{
if (Stack->List == NULL)
{
Stack->List = NewNode;
}
else
{
//최상위 노드를 찾아 NewNode를 쌓는다.(연결)
Node* OldTop = Stack->List;
while (OldTop->Next != NULL)
{
OldTop = OldTop->Next;
}
OldTop->Next = NewNode;
}
//스택의 Top 필드에 새 노드의 주소를 등록.
Stack->Top = NewNode;
}
int LLS_GetSize(LLS* Stack)
{
int Count = 0;
Node* Current = Stack->List;
while (Current != NULL)
{
Current = Current->Next;
Count++;
}
return Count;
}
Node* LLS_Top(LLS* Stack)
{
return Stack->Top;
}
|
2.LinkedList_Stack.c
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
|
int main(void)
{
unsigned int Stack_Size = 0;
LinkedStack* Stack;
Node* Popped;
LLS_CreateStack(&Stack);
LLS_Push(Stack, LLS_CreateNode("abc"));
LLS_Push(Stack, LLS_CreateNode("def"));
LLS_Push(Stack, LLS_CreateNode("ghi"));
Popped = LLS_Pop(Stack);
Stack_Size = LLS_GetSize(Stack);
printf(" Size : %d, Top :%s\n\n", Stack_Size, LLS_Top(Stack)->Data);
printf("Popped : %s\n", Popped->Data);
return 0;
}
|
3. 실행 화면
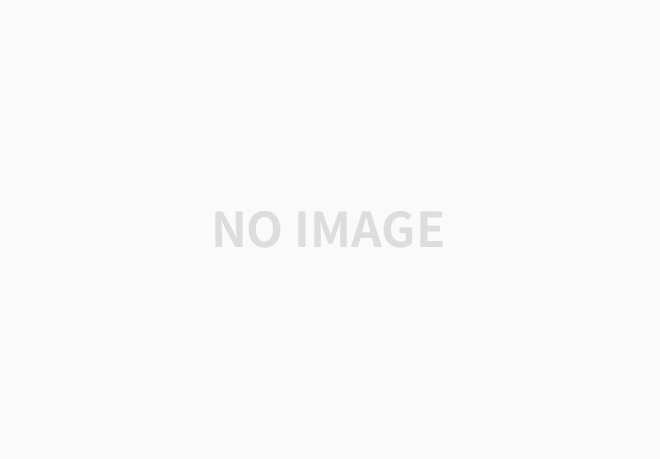
300x250
'DataStructure' 카테고리의 다른 글
이중 연결 리스트 -Double Linked List(2) (삭제, 삽입, 생성,추가) - C 언어 (0) | 2019.06.03 |
---|---|
단일 연결 리스트 -Linked List(2) (노드탐색, 삭제, 삽입) - C 언어 (0) | 2019.04.10 |
단일 연결 리스트 -Linked List(1) (노드생성, 삭제, 추가) - C 언어 (0) | 2019.04.07 |
댓글